Array
Let’s say you are creating a Beat Saber game, and you need to keep track of when each block should appear during each music. We could create a variable for each block with the timestamp of its appearance, like so:
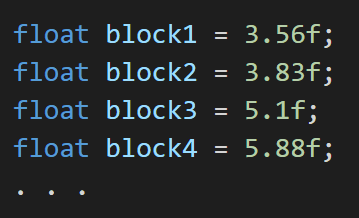
But that is simply impossible, especially with some music having over 1 thousand blocks. For these kinds of problems, C# has Arrays, “containers” that store multiple data sequentially. So when you are dealing with enemies, quests, targets, etc., you will probably use arrays or some similar data structure.
Strings are a special type of arrays, as they are a sequential collection of characters. But due to their frequent usage, C# provides a special built-in way to use them.
Building Arrays
As we said, arrays store multiple data, but to understand how to access that data, arrays follow some rules:
Arrays can only store the same kind of data, i.e., they share the same DataType.
Array elements are stored sequentially for easy data access.
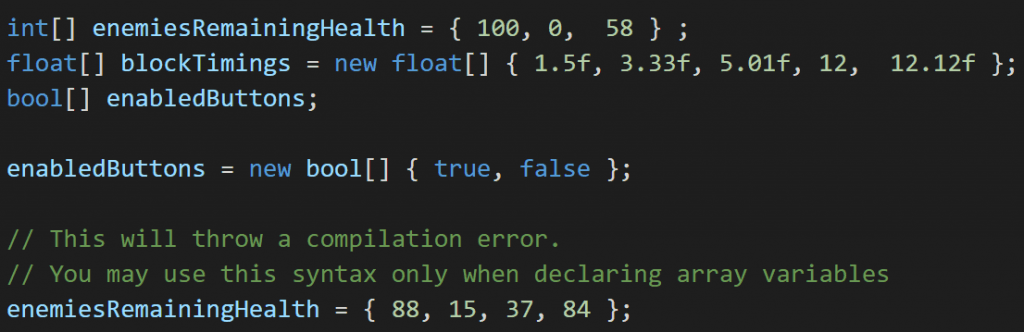
You declare array variables much like any other variable, set a DataType, add the square brackets “[ ]” indicating this is an array, and finish it with the variable name. And just like any other variable, you can assign a value to it upon declaration or re-assign a value later.
The values stored in the array are declared inside the curly brackets “{ }”; they are added to the collection in the same order declared, and must fit the DataType of the array.
The new keyword will be explained in detail in the next chapter, for now think of it as if you were instantiating the array, “allocating space” for the whole “collection”.
Accessing Array Items
Like I said earlier, arrays store the data sequentially, and so, each entry can be accessed through an index:
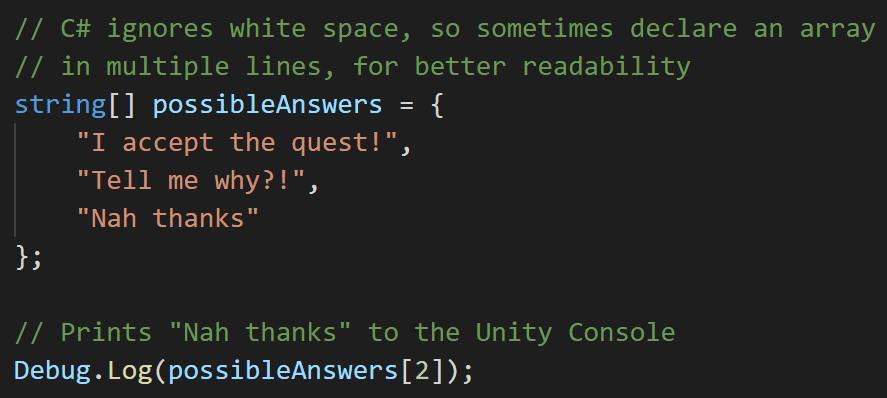
In C#, the arrays are indexed starting from 0, that is why the previous example prints “Nah thanks” instead of “Tell me Why?!” when using the index: 2.
Editing Arrays
Remember when I said the new keyword was to separate space for the array? This means we can’t go changing an array size willy nilly. So if you want to alter the size of an array, refer back to the Building arrays section and re-assign the array variable by creating a new array.
Changing the values stored in an array, on the other hand, is definitely possible! 🙂
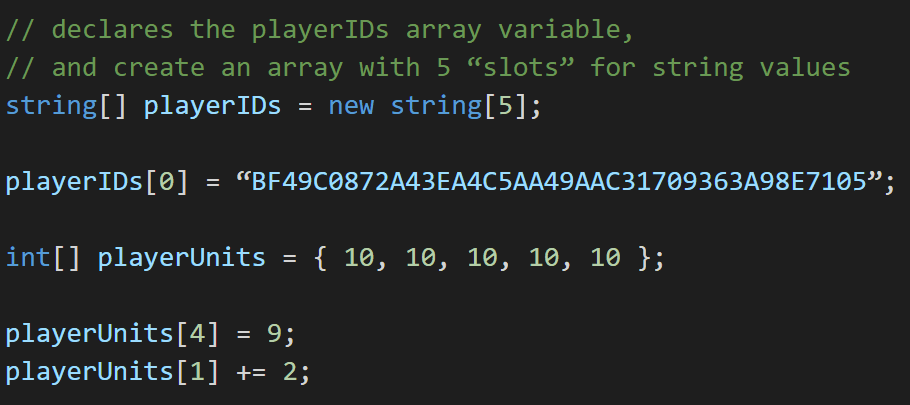
Using values inside an array is really similar to any other variable; just make sure to add which index of the array you are modifying, and you are good to go!
When you declare an array and provide only its size, as we just did for “playerIDs”, each array entry is filled with the default value of the array DataType:
int, float = 0
bool = false;
string = null;
Null values will also be covered in the next lesson. For now, think of it like this: Remember that strings are arrays? When an array has a null value, it is because we still haven’t “allocated any space” for it, and so it is like it doesn’t exist.
Built-In Functionality
Arrays come with some built-in functionality for the most common operations done with arrays. I will cover the main ones, but you can find an extensive list in the Microsoft documentation.
Array Length
Knowing the size of an array is extremely useful, especially when declaring arrays with sizes that vary per execution.
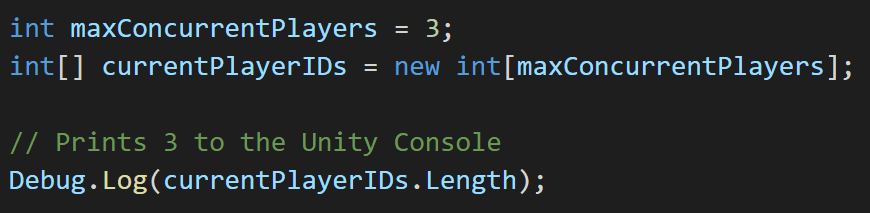
Be careful not to try to access or modify values outside of the array's bounds; doing so will give you an error: “IndexOutOfRangeException: Index was outside the bounds of the array.”
This is the Array.Length documentation for more info.
IndexOf Method
Next on the list is the Array.IndexOf method. This will search the provided array for a target value and return the index of the first occurrence, if there is one.
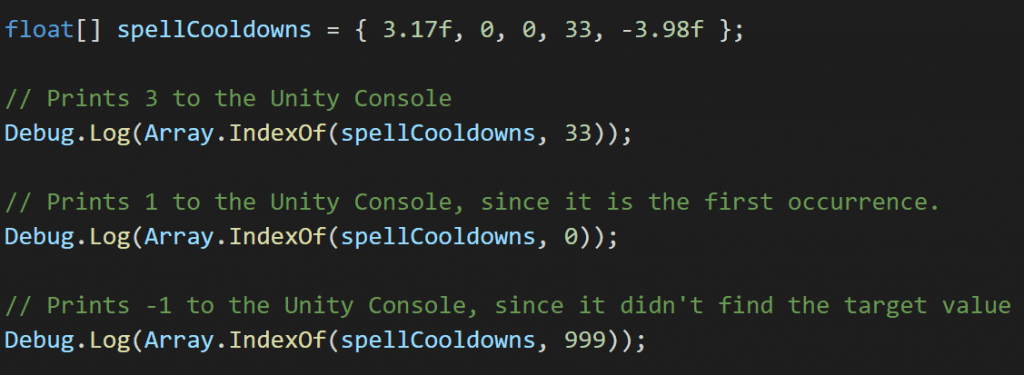
There are some overloads to this method, where you can provide a range for the search; make sure to check the documentation 🙂
Sort Method
Next up, Array.Sort! Oftentimes you need to set an array value in a specific order, for that we have the Sort method.

Number arrays are sorted in ascending order (lowest to highest), while string arrays are sorted alphabetically. There are ways to change the sorting logic using overrides of this function; it uses more advanced techniques, but definitely take a look at the documentation if you ever need it!
Loops
So far we can store multiple data in arrays, but still, if we were to actually process that data, we still need to do it manually, one by one. And that is where loops come into play!
I won’t cover while and do..while loops here, because they are not used too much, and usually can be rewritten in terms of for and foreach loops.
For Loop
The for loop is used when you want to execute a block of code an X number of times. It follows the following syntax:
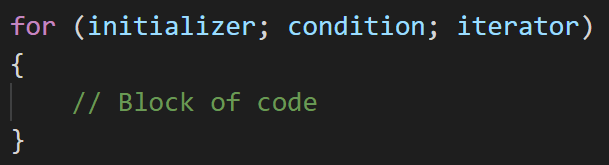
Initializer
This is executed only once, before anything else, and it is mostly used to declare a variable that will be used during the iteration.
Condition
This statement must be a boolean expression. Before every loop, this expression is executed; if the condition evaluates to true, the loop keeps executing; otherwise, it stops, and the control flow proceeds to the next statement after the for loop.
Iterator
The iterator statement executes after each loop, that is after the condition and block of code are executed. It is most commonly used for incrementing the variable declared in the initializer.
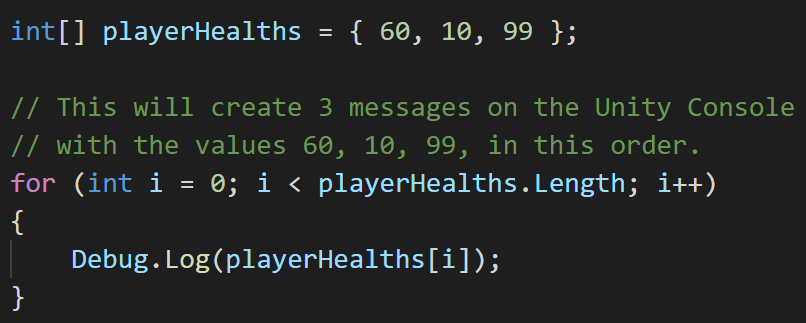
It is a common practice to name the iterator variable “i” or “j” when “i” is already in use. The iterator variable is only accessible inside the for block.
Tip: “i++” is equivalent to “i += 1”.
For Each Loop
For each loop is a more readable way to write for loops. It is used when you don’t care about the values indexes, but only about the values themselves. It follows this syntax:
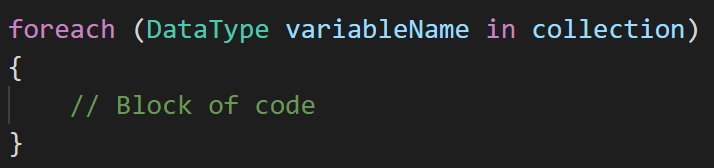
The for each will iterate in order over the entire collection provided, and on each iteration, set the variable “variableName” to the current value of the iteration. Make sure the DataType provided matches the DataType of the values inside the collection.
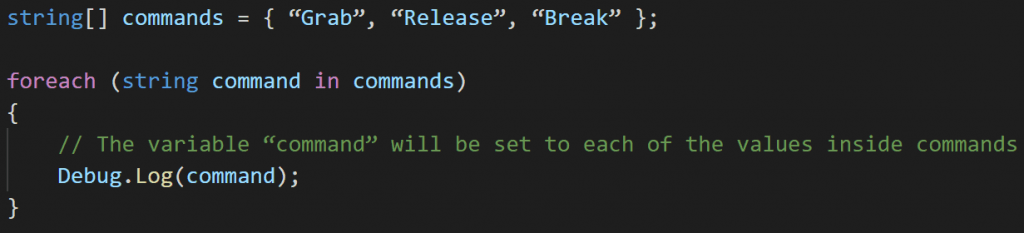
For now, you only know arrays, but for each loops can be used with other collection structures as well, like Dictionaries and Lists, they use some more advanced features but are worth a look.
Coming up next!
Next week we’ll take a look at the heavily awaited Classes and Objects. With all the logic aspects out of the way, it’s time to see how it all fits together.
Happy coding 🙂 🔙 Back to the Learn C# for Unity menu